Mindset shift: Module driven development
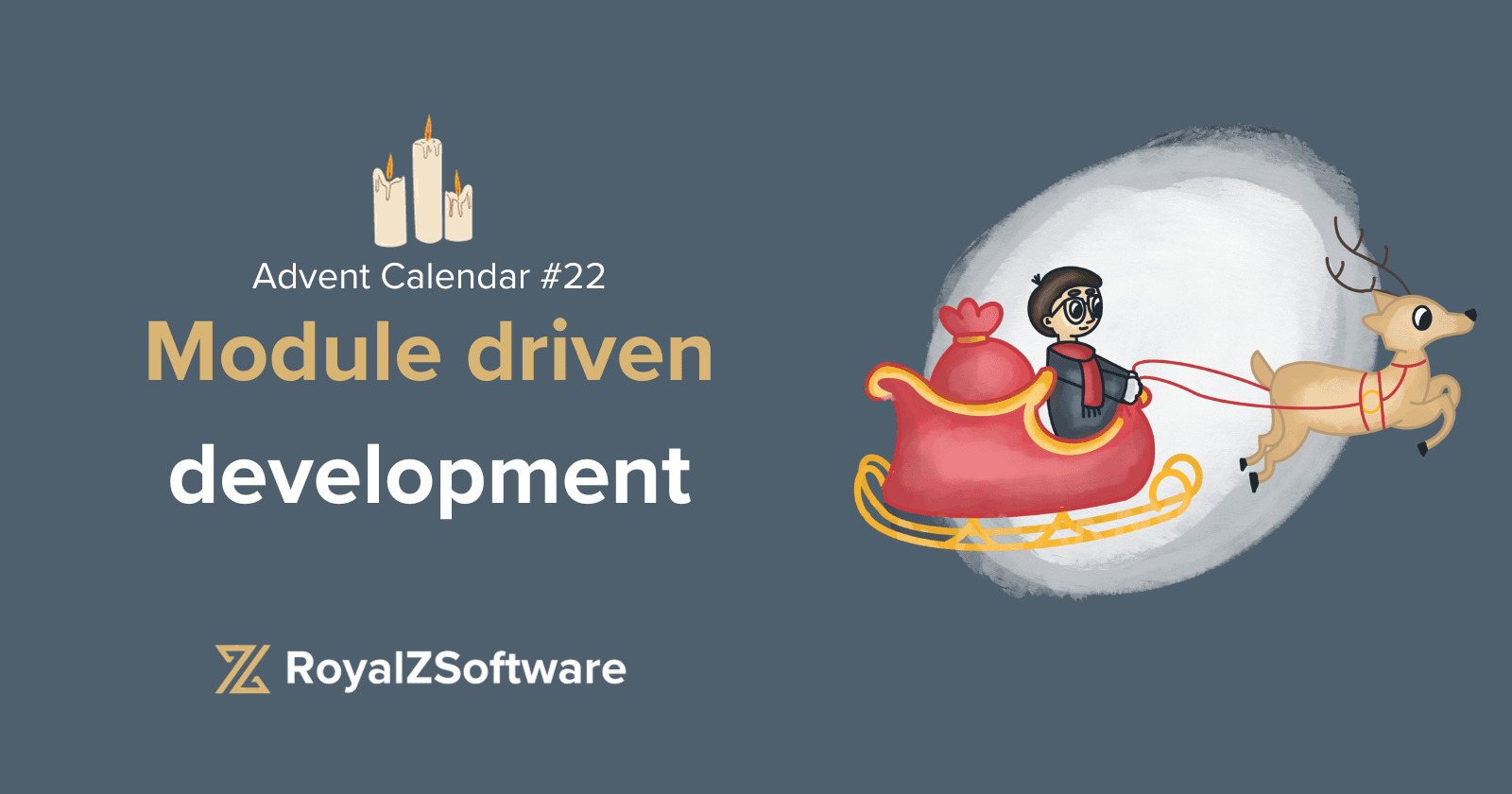
Once I shifted my mindset to module driven development, my code got way cleaner.
What is a module?
Modules or packages are a composition of functionality that accomplish one specific goal. They might have dependencies to other modules.
How to develop module driven?
Think about the functionality that your module should provide and make a clear cut. Think about the public API (how the functionality is embedded into an application) and then start implementing, with a clear scope in mind.
It's easy to get lost in adding functionality over and over to a later gigantic project. That's when things get messy.
After that, use the finished written module in your greater application scope.
Actual examples
Angular
Angular gives a great example on how to work with modules. Everything there is called a module (or nowadays a standalone component).
Angular Modules can provide certain classes to interact with the components. They also provide the components themselves.
Let's say we have a GreetModule
. The feature scope contains:
-
a
GreetComponent
that receives the name of the person to greet via a text input field -
a
NotifyService
that spawns analert()
dialog for the greeting
Instead of putting all the code into the actual AppModule
, we create a GreetModule
, which provides the NotifyService
, and (declares AND exports) the GreetComponent
. That way it can be used outside of the module.
Why should I do this? For reusability?
While in fact reusability is a great benefit, it's not the primary goal of this.
It's mostly encapsulation. If you are encapsulating your code within a separate module, you are not running into issues with other parts of your software, when changing this module.
Or at least, you can find out easily what other code depends on your module.